VBA Call / Run Sub From another Subroutine
Written by
Reviewed by
In this Article
This tutorial will teach you how to call a sub procedure from within another sub procedure in VBA
It is very useful to write code that can be used repetitively, and called from multiple sub procedures in your project – it can save an enormous amount of time and makes the VBA code far more efficient.
Running a Sub Procedure from another Sub Procedure
Consider the 3 Sub Procedures below:
Sub TestRoutine()
RunRoutine1
RunRoutine2
End Sub
Sub RunRoutine1()
MsgBox "Good Morning"
End Sub
Sub RunRoutine2()
MsgBox "Today's date is " & Format(Date, "mm/dd/yyyy")
End Sub
If we run the Sub Procedure – TestRoutine – it will call RunRoutine1 and RunRoutine2 and 2 message boxes will appear.
There is no limit to the number of Sub Procedures you can call from another Sub Procedure.
Using the Call Statement
You can also use the Call Statement in front of the procedure name, to make your code easier to read. However, it has no effect whatsoever on how the code is run or stored.
Sub TestRoutine()
Call RunRoutine1
Call RunRoutine2
End Sub
Calling a Sub with Arguments
It is also possible to call a sub with arguments
Sub TestRoutine()
RunRoutine1 ("Melanie")
RunRoutine2 ("Have a lovely Day")
End Sub
Sub RunRoutine1(strName as String)
MsgBox "Good Morning " & " & strName
End Sub
Sub RunRoutine2(strMessage as String )
MsgBox "Today's date is " & Format(Date, "mm/dd/yyyy") & VbCrLf & strMessage
End Sub
Calling a Sub with Named Arguments
If you name your arguments, you don’t have to pass them in the same order to your sub routines.
Sub TestRoutine()
RunRoutine1 strGreeting:="How are you?", strName:="Melanie"
End Sub
Sub RunRoutine1(strName as String, strGreeting as string
MsgBox "Good Morning " & " & strName & vbCrLf & strGreeting
End Sub
VBA Coding Made Easy
Stop searching for VBA code online. Learn more about AutoMacro - A VBA Code Builder that allows beginners to code procedures from scratch with minimal coding knowledge and with many time-saving features for all users!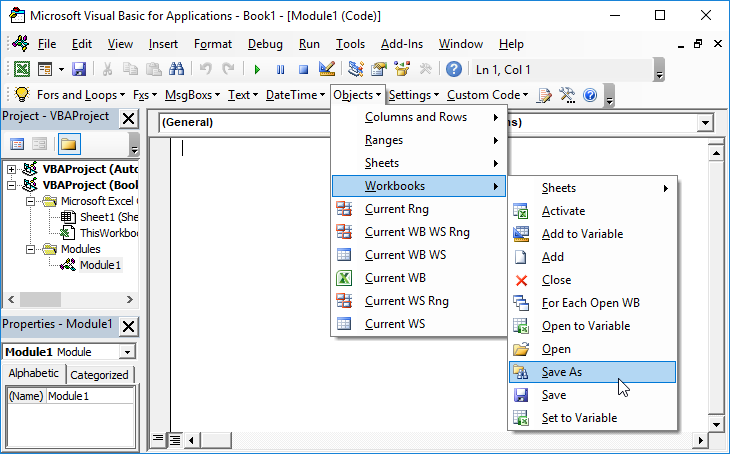
Learn More!